Railsで画像アップロード機能を簡単に実装していきます。
今回はGemファイルへ記述するので簡単に実装できます。
ほぼコピペだけで実装可能です。
目次
Gemファイルへ追加する
追加するGemは2つ
gem 'carrierwave'
gem 'mini_magick'
このファイルを追加するだけでOKです。
ただし、mini_magickを使うには ImageMagickをサーバーにインストールする必要があります。
ImageMagick をサーバーへインストール
もしImageMagickがサーバーに入ってない場合はインストールしましょう。入っているかの確認は
$ convert -version
このコマンドで入っているか確認できます。
ImageMagickが入っいる場合は
Version: ImageMagick 6.9.7-4 Q16 x86_64 20170114 http://www.imagemagick.org
Copyright: © 1999-2017 ImageMagick Studio LLC
このような感じで表示されます。
ImageMagickが入ってない場合は
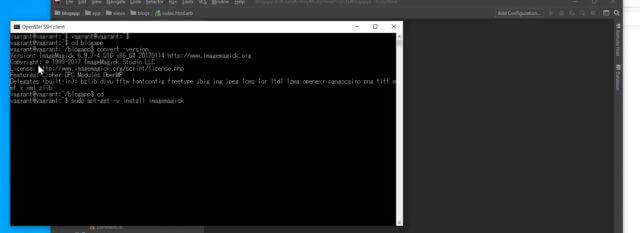
$ sudo apt-get -y install imagemagick
でUbuntuの場合は上記のコマンドでインストールできますので安心してください。
- Macの場合は
$ brew install imagemagick - CentOSの場合は
$ sudo yum install imagemagick
Gemをインストールします
$ bundle
画像アップロードできるページを作成
$ rails g scaffold upload image:string title:text body:string
$ rails g uploader images
$ rails db:migrate
modelsファイルの修正
class Upload < ApplicationRecord
mount_uploader :image, ImagesUploader
end
コントローラーファイルは確認でOK
class UploadsController < ApplicationController
before_action :set_upload, only: [:show, :edit, :update, :destroy]
# GET /uploads
# GET /uploads.json
def index
@uploads = Upload.all
end
# GET /uploads/1
# GET /uploads/1.json
def show
end
# GET /uploads/new
def new
@upload = Upload.new
end
# GET /uploads/1/edit
def edit
end
# POST /uploads
# POST /uploads.json
def create
@upload = Upload.new(upload_params)
respond_to do |format|
if @upload.save
format.html { redirect_to @upload, notice: 'Upload was successfully created.' }
format.json { render :show, status: :created, location: @upload }
else
format.html { render :new }
format.json { render json: @upload.errors, status: :unprocessable_entity }
end
end
end
# PATCH/PUT /uploads/1
# PATCH/PUT /uploads/1.json
def update
respond_to do |format|
if @upload.update(upload_params)
format.html { redirect_to @upload, notice: 'Upload was successfully updated.' }
format.json { render :show, status: :ok, location: @upload }
else
format.html { render :edit }
format.json { render json: @upload.errors, status: :unprocessable_entity }
end
end
end
# DELETE /uploads/1
# DELETE /uploads/1.json
def destroy
@upload.destroy
respond_to do |format|
format.html { redirect_to uploads_url, notice: 'Upload was successfully destroyed.' }
format.json { head :no_content }
end
end
private
# Use callbacks to share common setup or constraints between actions.
def set_upload
@upload = Upload.find(params[:id])
end
# Never trust parameters from the scary internet, only allow the white list through.
def upload_params
params.require(:upload).permit(:image, :title, :body)
end
end
今回は自動で生成された状態のままでOKです。
uploadersファイルの編集
class ImagesUploader < CarrierWave::Uploader::Base
# Include RMagick or MiniMagick support:
# include CarrierWave::RMagick
include CarrierWave::MiniMagick
# Choose what kind of storage to use for this uploader:
storage :file
# storage :fog
# Override the directory where uploaded files will be stored.
# This is a sensible default for uploaders that are meant to be mounted:
def store_dir
"uploads/#{model.class.to_s.underscore}/#{mounted_as}/#{model.id}"
end
def extension_whitelist
%w(jpg jpeg gif png)
end
process :resize_to_limit => [700, 700]
version :thumb do
process resize_to_fill: [100, 100]
end
end
uploadersファイルは画像をアップロードするための設定ファイルになります。
ここで設定した内容で出力されるようになります。
デザインを変更していきます
Viewsファイルを確認
<%= form_with(model: upload, local: true) do |form| %>
<% if upload.errors.any? %>
<div id="error_explanation">
<h2><%= pluralize(upload.errors.count, "error") %> prohibited this upload from being saved:</h2>
<ul>
<% upload.errors.full_messages.each do |message| %>
<li><%= message %></li>
<% end %>
</ul>
</div>
<% end %>
<div class="field">
<%= form.label :image %>
<%= form.file_field :image %>
</div>
<div class="field">
<%= form.label :title %>
<%= form.text_field :title %>
</div>
<div class="field">
<%= form.label :body %>
<%= form.text_area :body %>
</div>
<div class="actions">
<%= form.submit %>
</div>
<% end %>
form.text_field :image になっているので form.file_field :image に変更します。
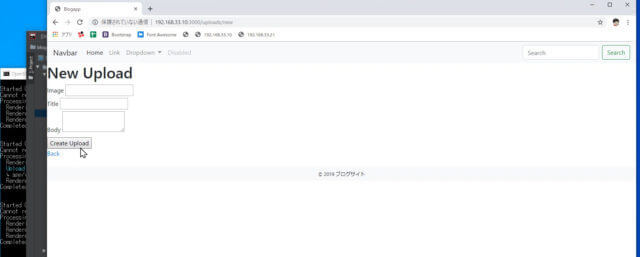
変更しないとこの様にインプットのフィールドになっています。
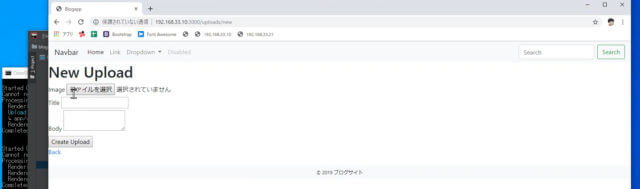
これでブラウザから画像を選択できるようになりました。
アップロード画像の表示サイズを変更する
<p id="notice"><%= notice %></p>
<p>
<strong>Image:</strong>
<%#= @upload.image %>
<%= image_tag @upload.image_url(:thumb ) if @upload.image.present? %>
</p>
<p>
<strong>Title:</strong>
<%= @upload.title %>
</p>
<p>
<strong>Body:</strong>
<%= @upload.body %>
</p>
<%= link_to 'Edit', edit_upload_path(@upload) %> |
<%= link_to 'Back', uploads_path %>
@upload.image_url()部分に :thumb を入れるとサムネイルサイズが表示されます。
通常のサイズ
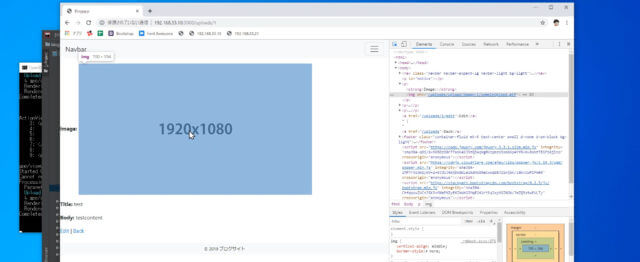
サムネイルサイズ
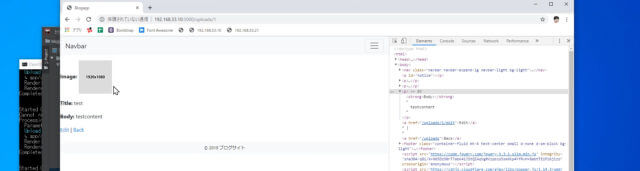
画像アップロード機能実装方法を動画で確認する
ここまでの流れを動画で確認できます。